API reference
Streamlit makes it easy for you to visualize, mutate, and share data. The API reference is organized by activity type, like displaying data or optimizing performance. Each section includes methods associated with the activity type, including examples.
Browse our API below and click to learn more about any of our available commands! 🎈
Display almost anything
st.write
Write arguments to the app.
st.write("Hello **world**!")
st.write(my_data_frame)
st.write(my_mpl_figure)
Magic
Any time Streamlit sees either a variable or literal value on its own line, it automatically writes that to your app using st.write
"Hello **world**!"
my_data_frame
my_mpl_figure
Text elements
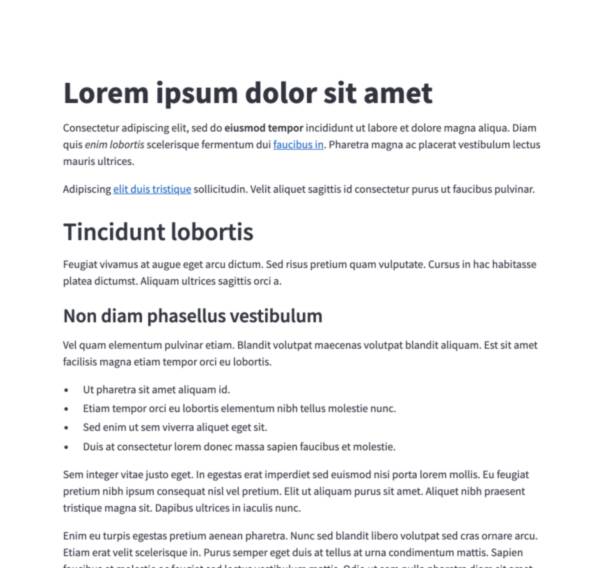
Markdown
Display string formatted as Markdown.
st.markdown("Hello **world**!")
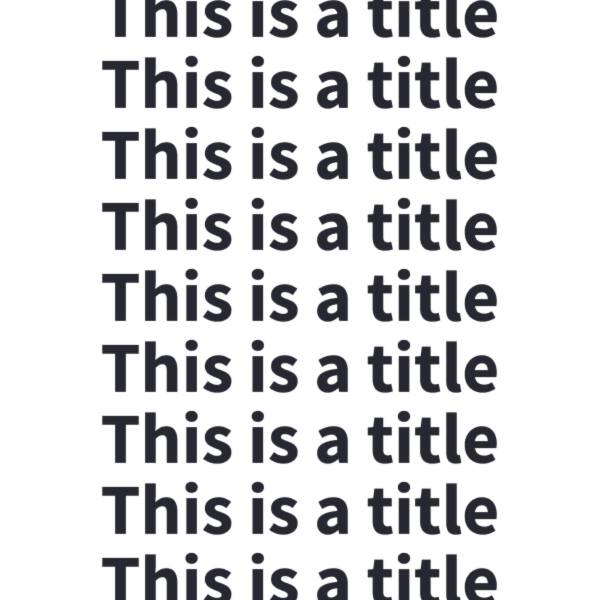
Title
Display text in title formatting.
st.title("The app title")
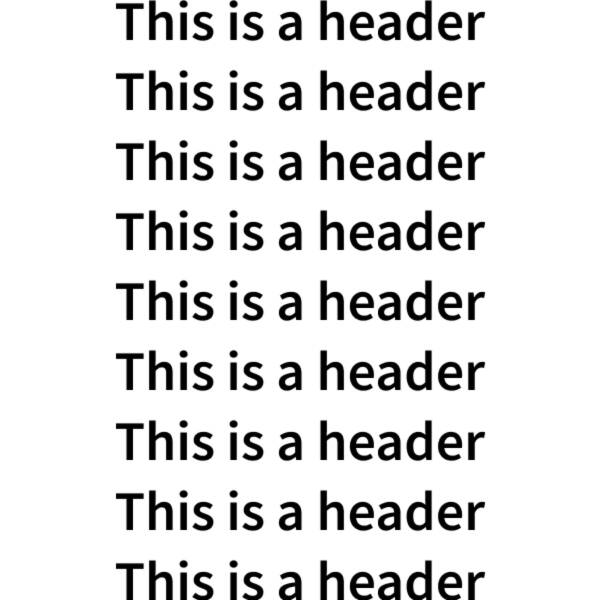
Header
Display text in header formatting.
st.header("This is a header")
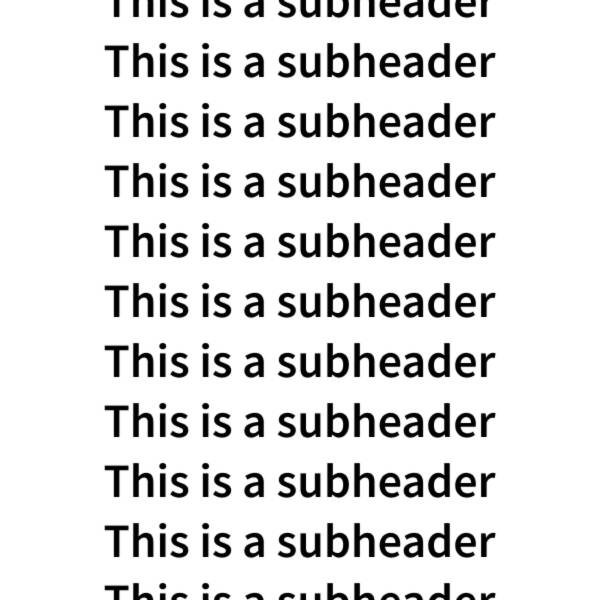
Subheader
Display text in subheader formatting.
st.subheader("This is a subheader")
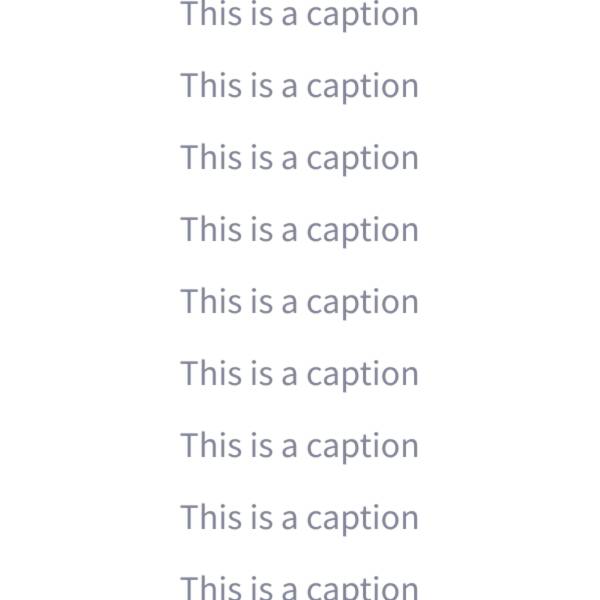
Caption
Display text in small font.
st.caption("This is written small caption text")
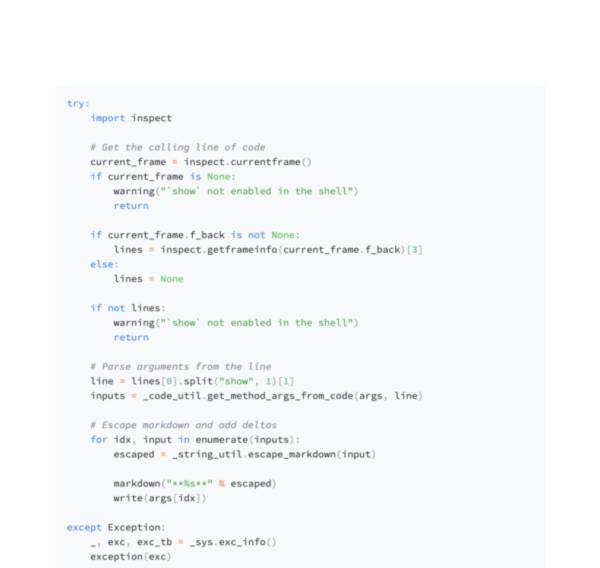
Code block
Display a code block with optional syntax highlighting.
st.code("a = 1234")
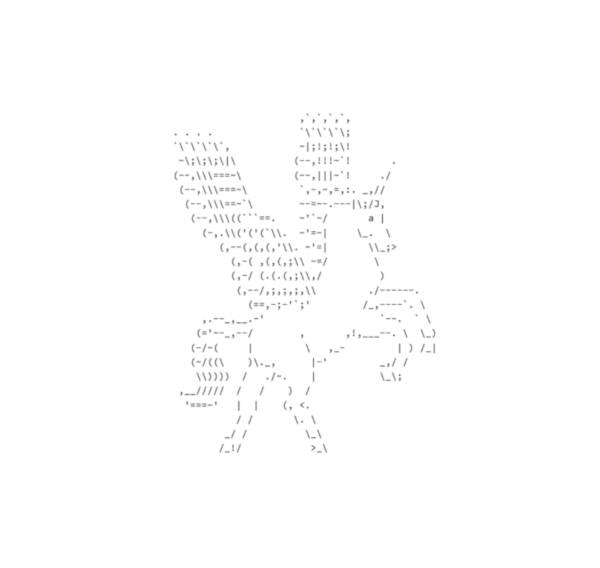
Preformatted text
Write fixed-width and preformatted text.
st.text("Hello world")
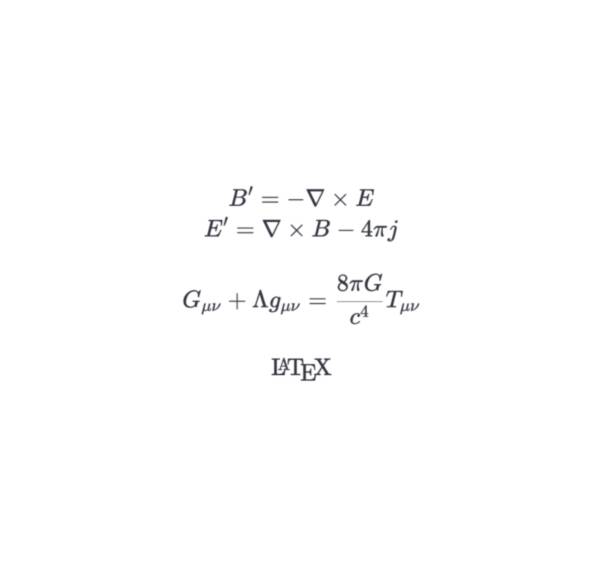
LaTeX
Display mathematical expressions formatted as LaTeX.
st.latex("\int a x^2 \,dx")
Data display elements
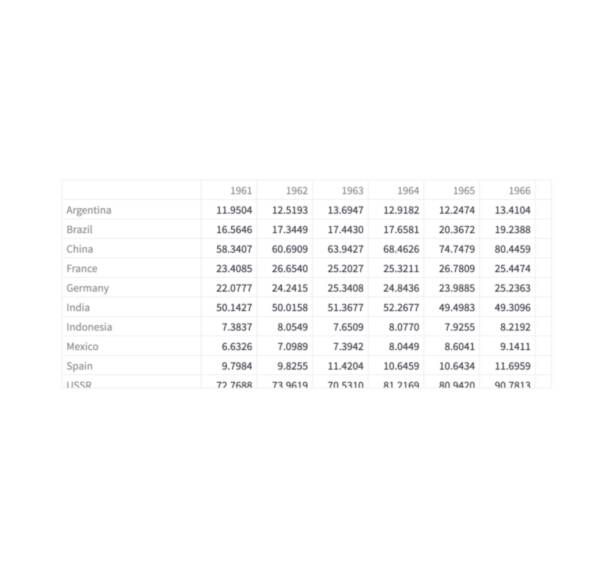
Dataframes
Display a dataframe as an interactive table.
st.dataframe(my_data_frame)
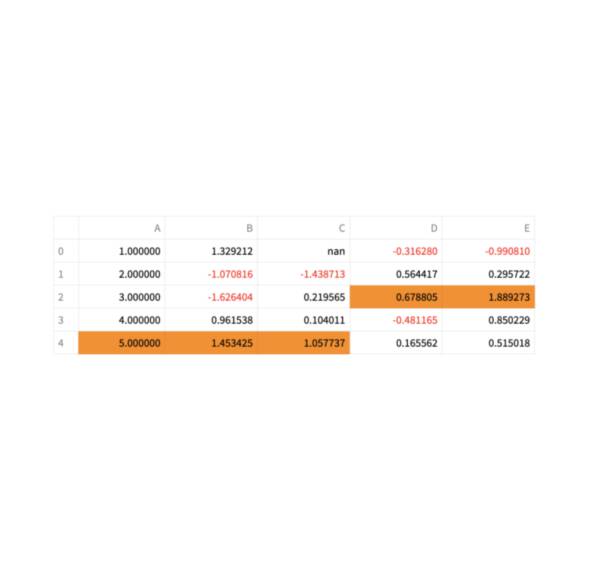
Static tables
Display a static table.
st.table(my_data_frame)
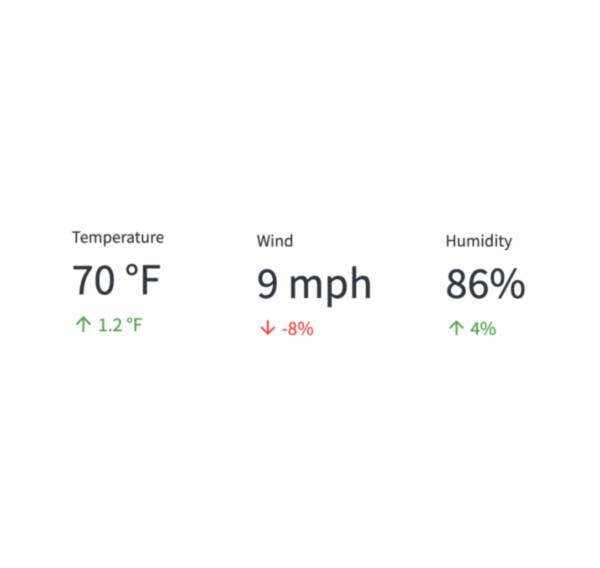
Metrics
Display a metric in big bold font, with an optional indicator of how the metric changed.
st.metric("My metric", 42, 2)
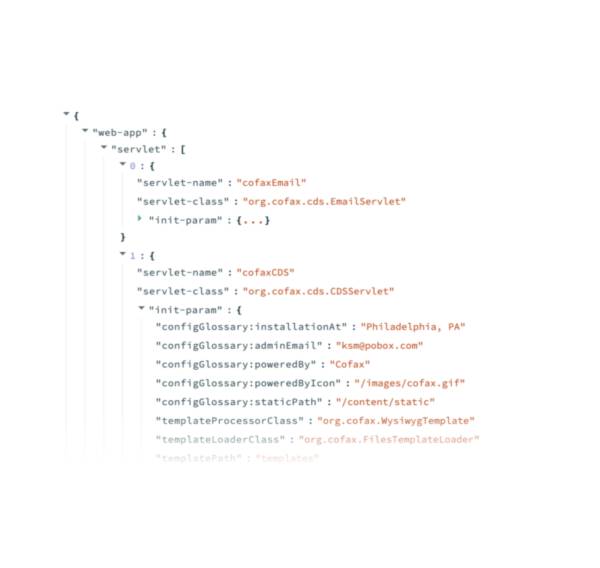
Dicts and JSON
Display object or string as a pretty-printed JSON string.
st.json(my_data_frame)
Chart elements
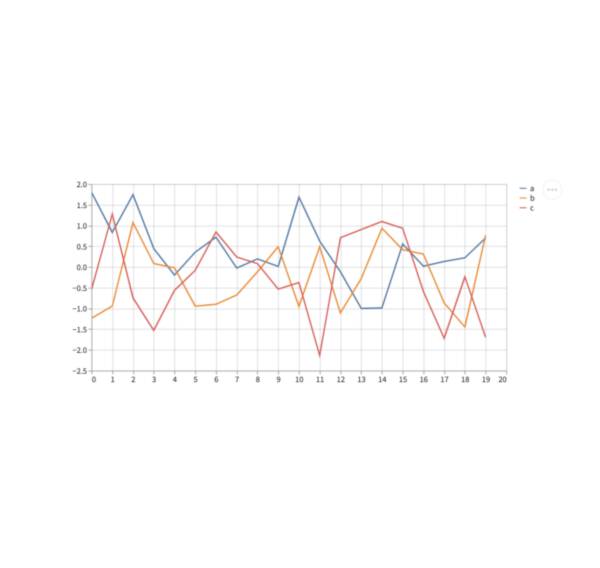
Simple line charts
Display a line chart.
st.line_chart(my_data_frame)
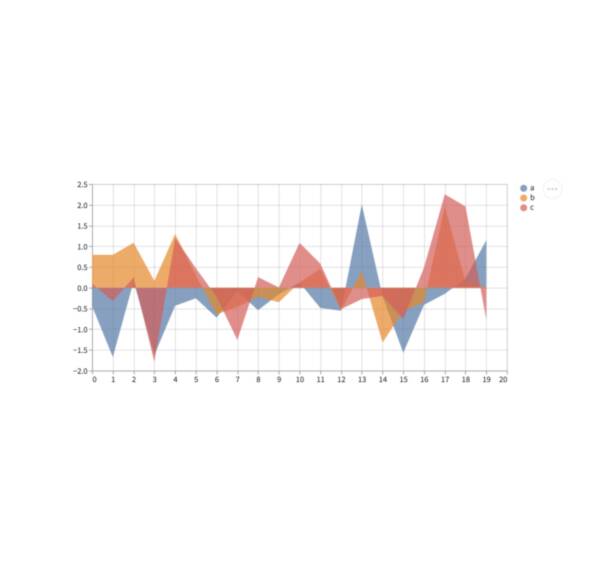
Simple area charts
Display an area chart.
st.area_chart(my_data_frame)
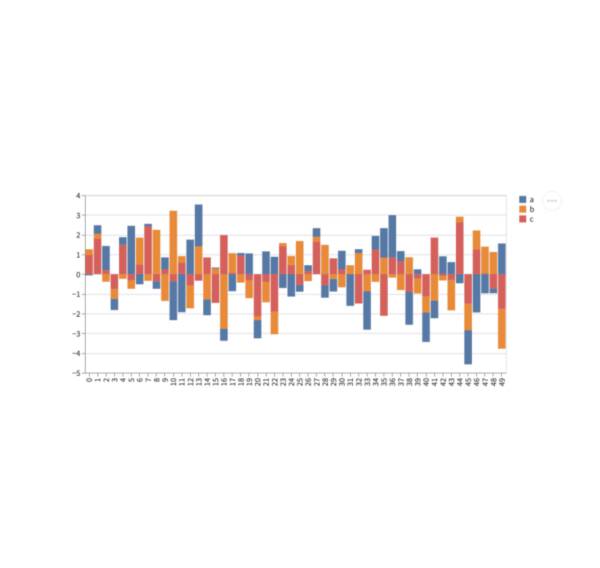
Simple bar charts
Display a bar chart.
st.bar_chart(my_data_frame)
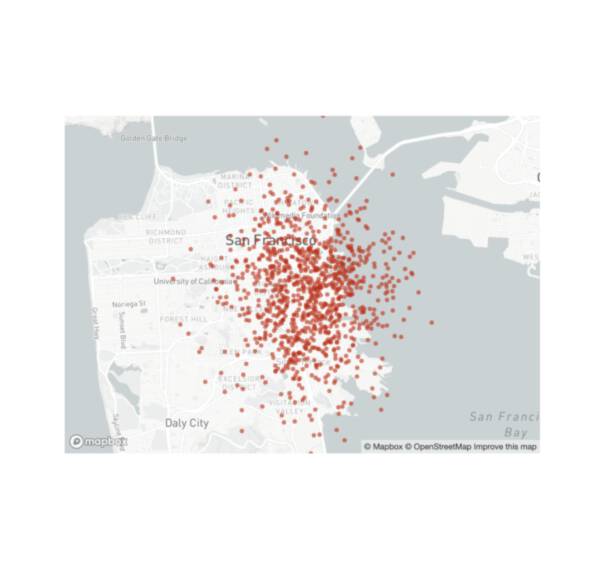
Scatterplots on maps
Display a map with points on it.
st.map(my_data_frame)
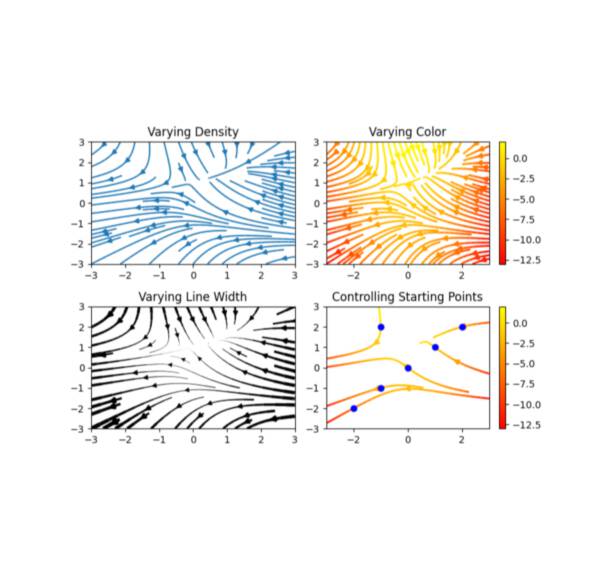
Matplotlib
Display a matplotlib.pyplot figure.
st.pyplot(my_mpl_figure)
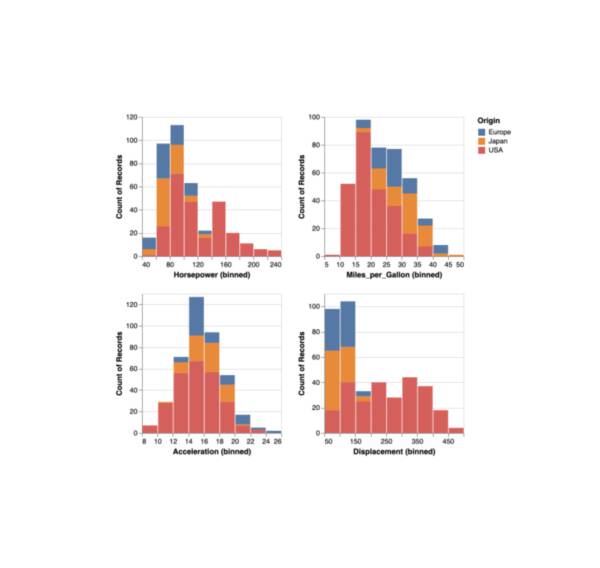
Altair
Display a chart using the Altair library.
st.altair_chart(my_altair_chart)
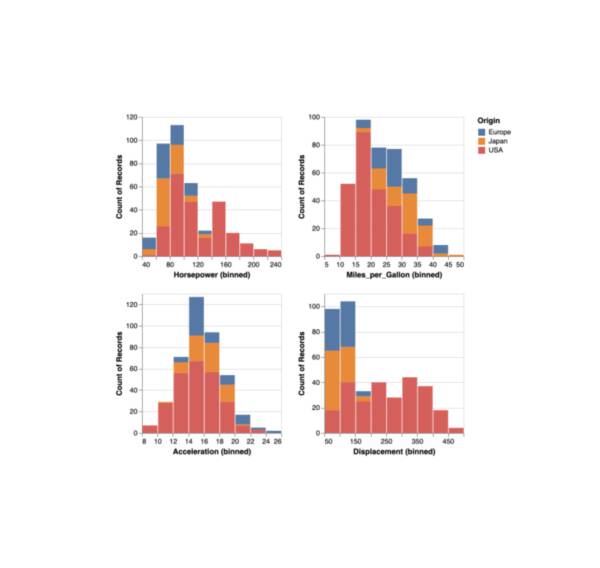
Vega-Lite
Display a chart using the Vega-Lite library.
st.vega_lite_chart(my_vega_lite_chart)
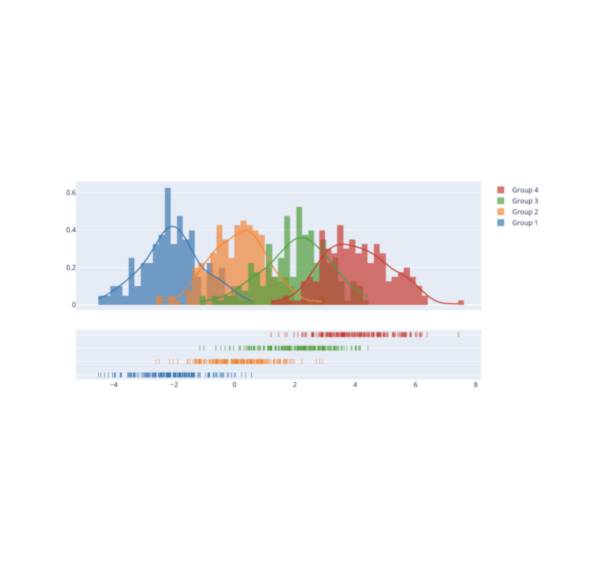
Plotly
Display an interactive Plotly chart.
st.plotly_chart(my_plotly_chart)
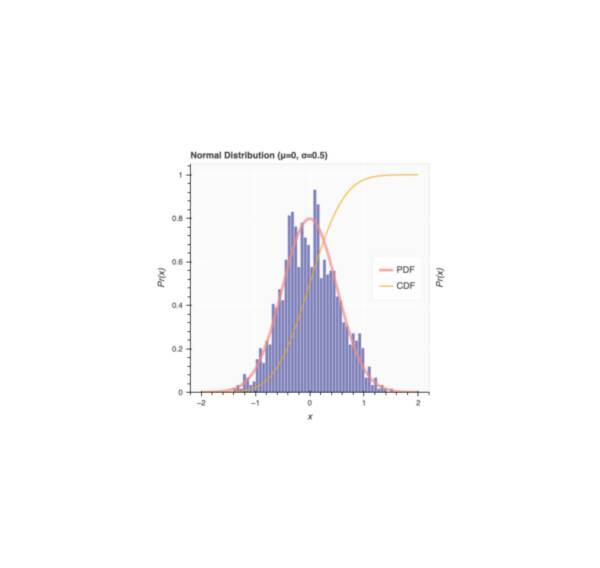
Bokeh
Display an interactive Bokeh chart.
st.bokeh_chart(my_bokeh_chart)
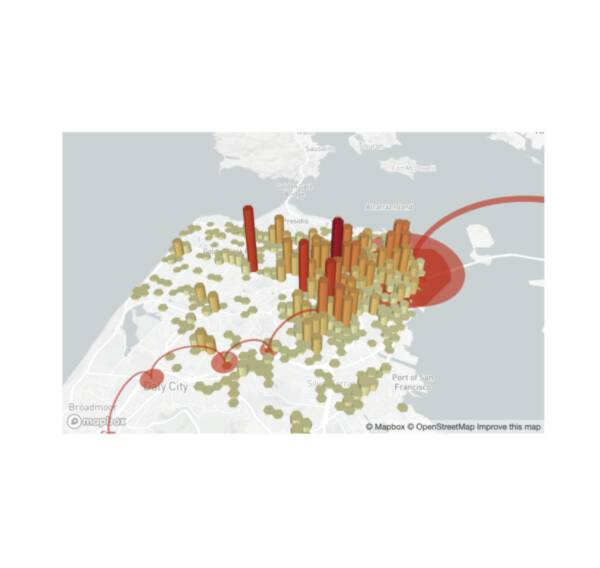
PyDeck
Display a chart using the PyDeck library.
st.pydeck_chart(my_pydeck_chart)
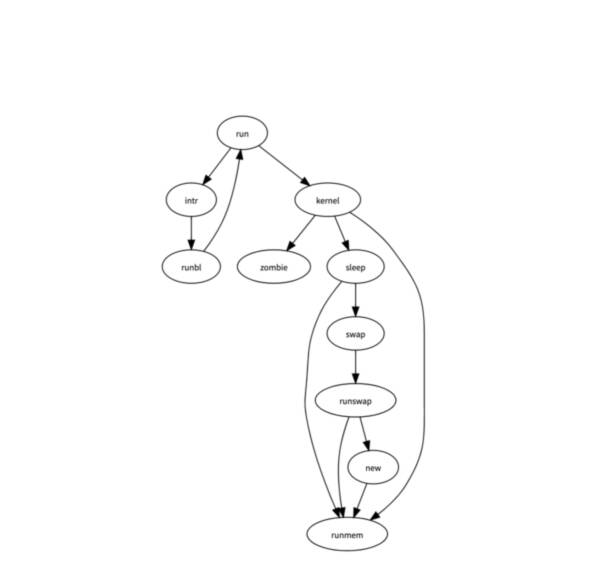
GraphViz
Display a graph using the dagre-d3 library.
st.graphviz_chart(my_graphviz_spec)
Input widgets
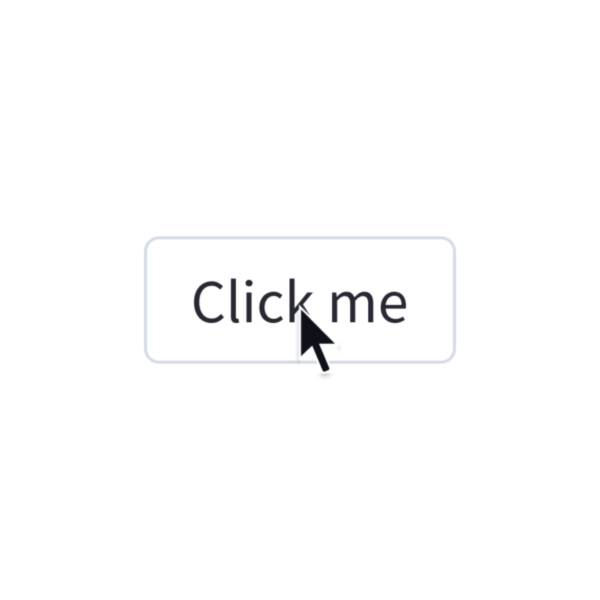
Button
Display a button widget.
clicked = st.button("Click me")
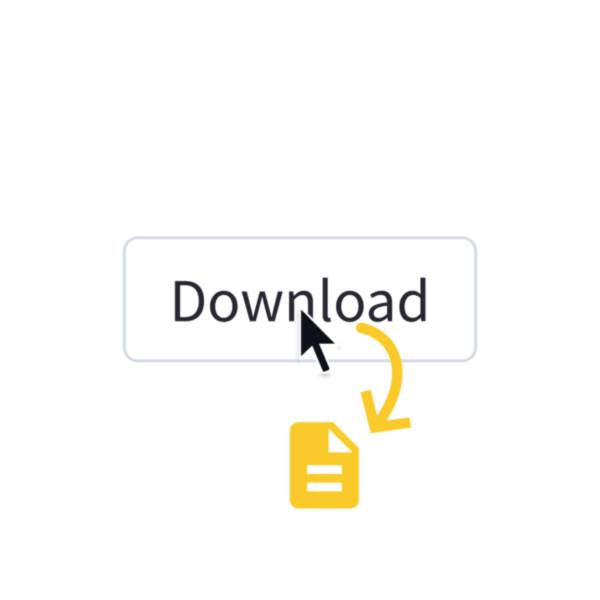
Download button
Display a download button widget.
st.download_button("Download file", file)
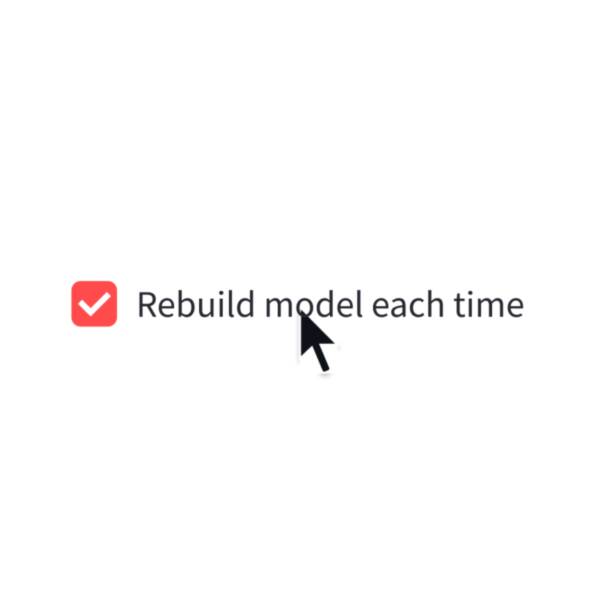
Checkbox
Display a checkbox widget.
selected = st.checkbox("I agree")
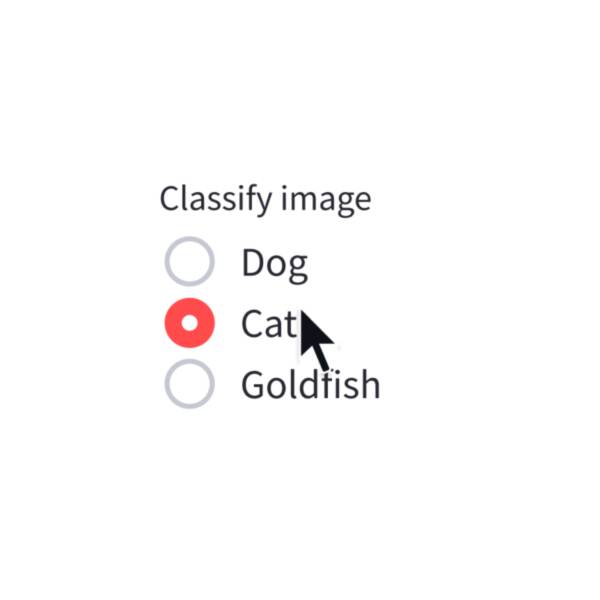
Radio
Display a radio button widget.
choice = st.radio("Pick one", ["cats", "dogs"])
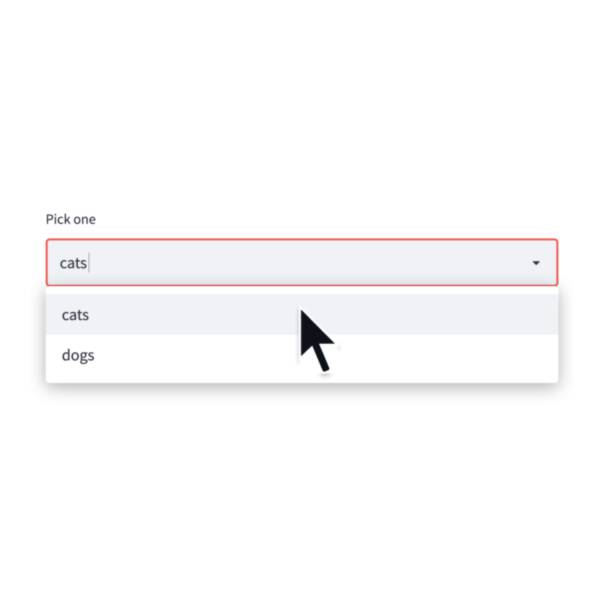
Selectbox
Display a select widget.
choice = st.selectbox("Pick one", ["cats", "dogs"])
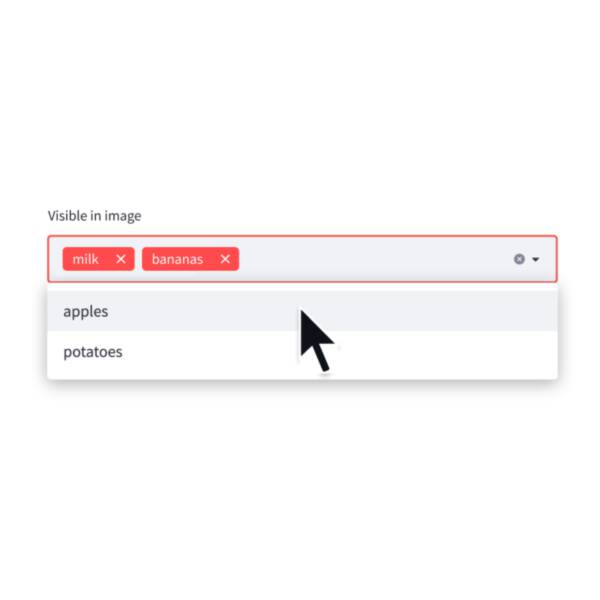
Multiselect
Display a multiselect widget. The multiselect widget starts as empty.
choices = st.multiselect("Buy", ["milk", "apples", "potatoes"])
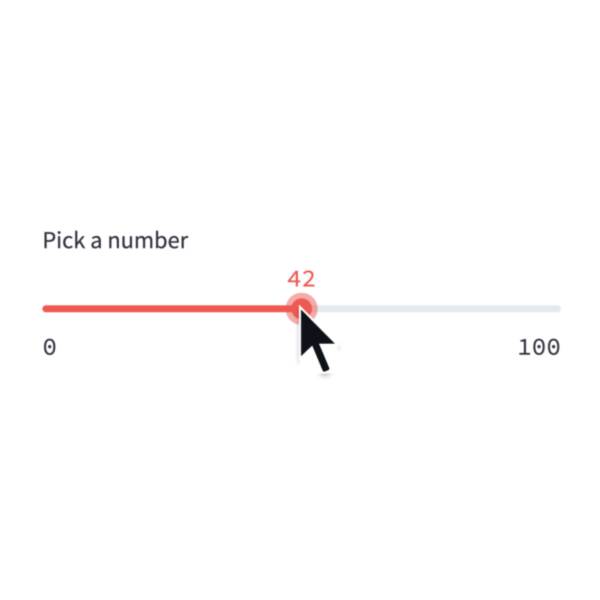
Slider
Display a slider widget.
number = st.slider("Pick a number", 0, 100)
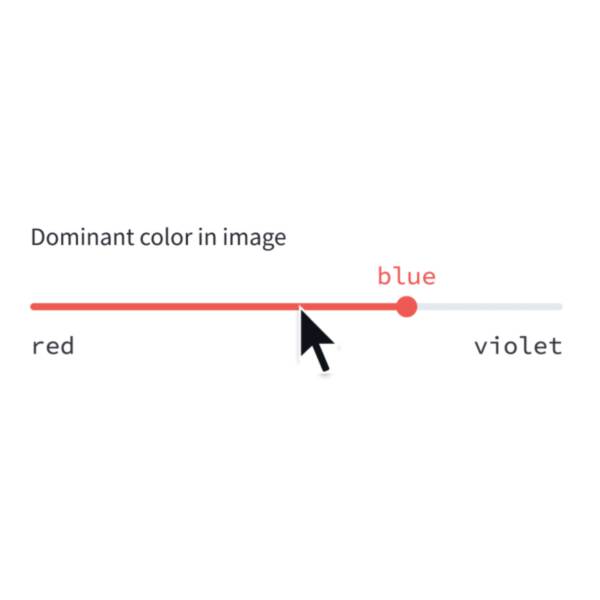
Select-slider
Display a slider widget to select items from a list.
size = st.select_slider("Pick a size", ["S", "M", "L"])
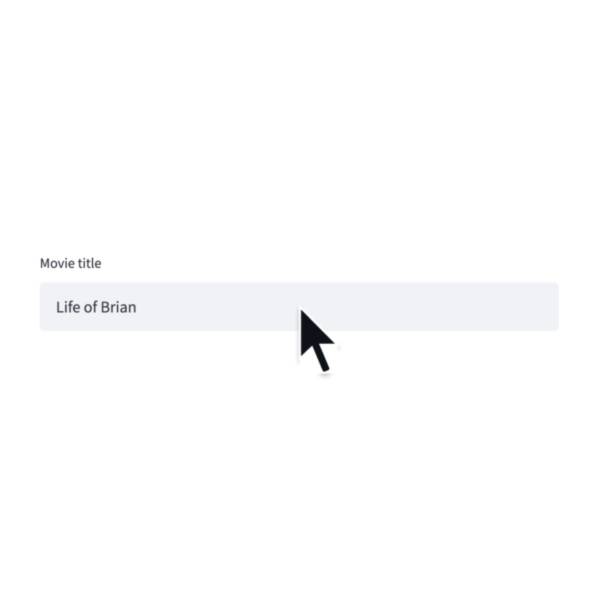
Text input
Display a single-line text input widget.
name = st.text_input("First name")
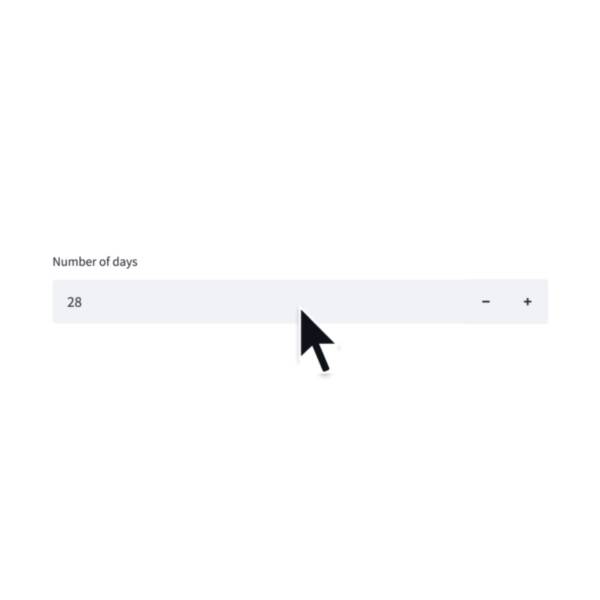
Number input
Display a numeric input widget.
choice = st.number_input("Pick a number", 0, 10)
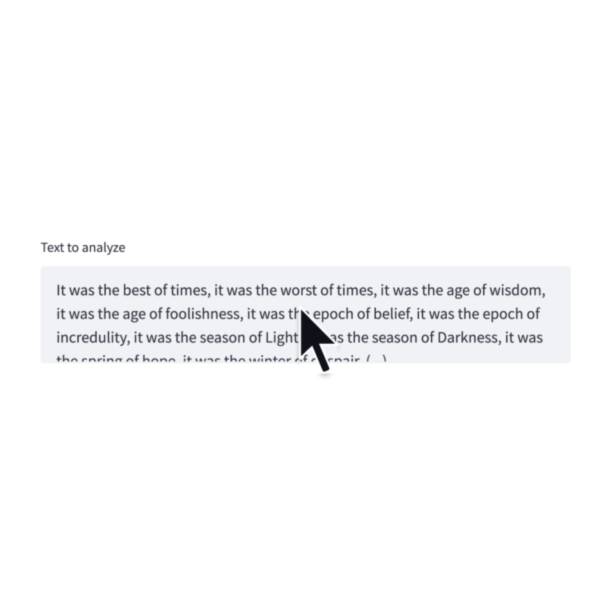
Text-area
Display a multi-line text input widget.
text = st.text_area("Text to translate")
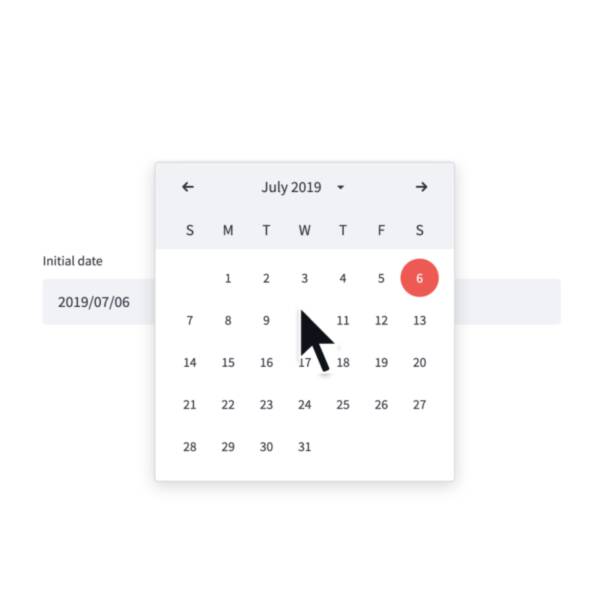
Date input
Display a date input widget.
date = st.date_input("Your birthday")
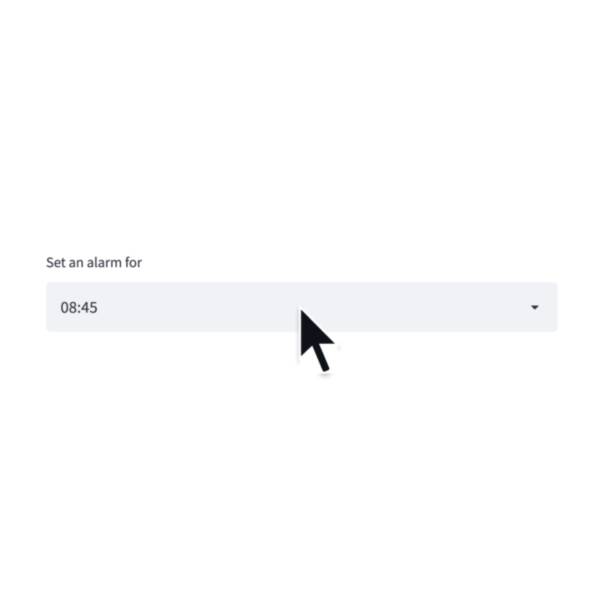
Time input
Display a time input widget.
time = st.time_input("Meeting time")
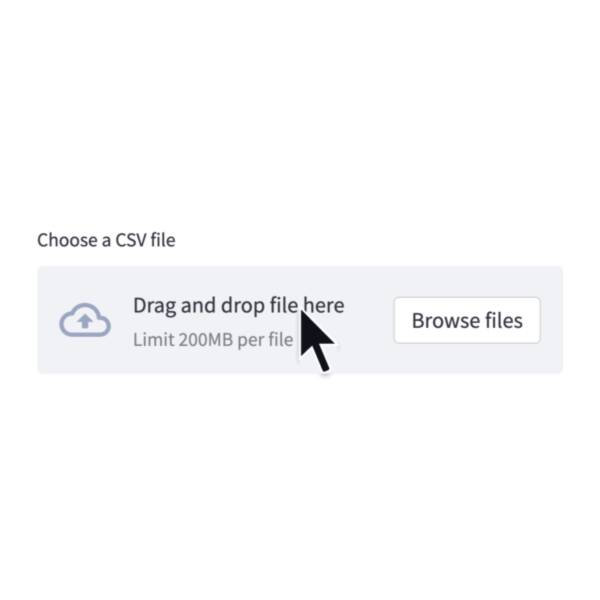
File Uploader
Display a file uploader widget.
data = st.file_uploader("Upload a CSV")
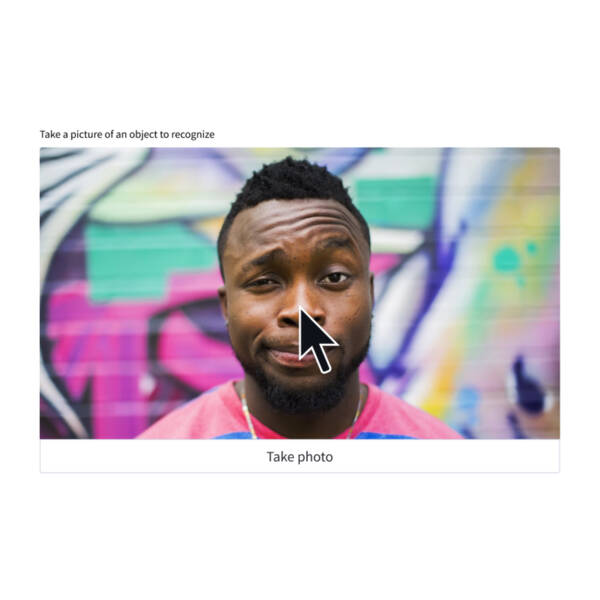
Camera input
Display a widget that allows users to upload images directly a camera.
image = st.camera_input("Take a picture")
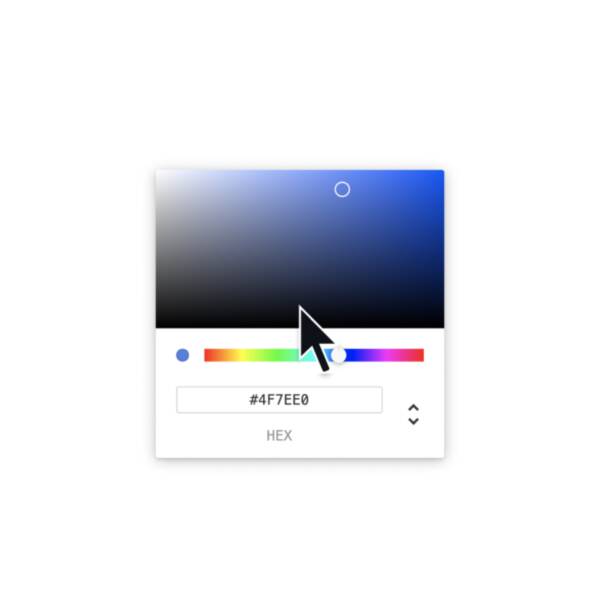
Color picker
Display a color picker widget.
color = st.color_picker("Pick a color")
Media elements
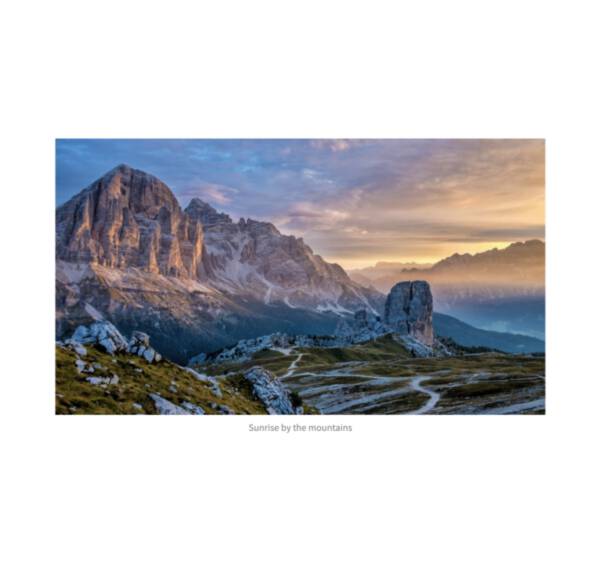
Image
Display an image or list of images.
st.image(numpy_array)
st.image(image_bytes)
st.image(file)
st.image("https://example.com/myimage.jpg")
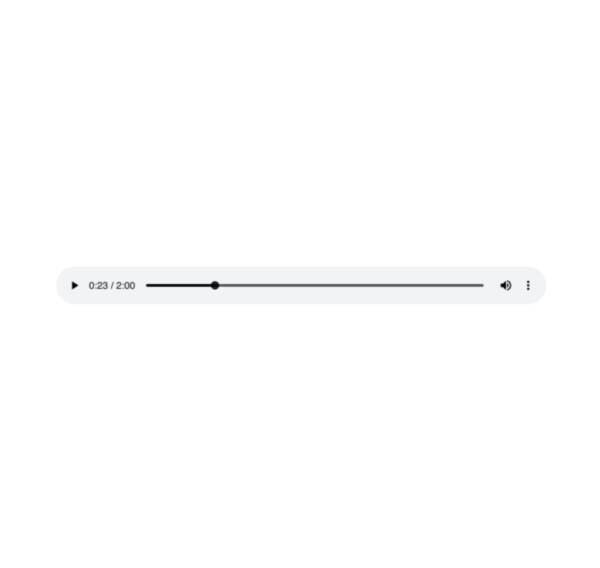
Audio
Display an audio player.
st.audio(numpy_array)
st.audio(audio_bytes)
st.audio(file)
st.audio("https://example.com/myaudio.mp3", format="audio/mp3")
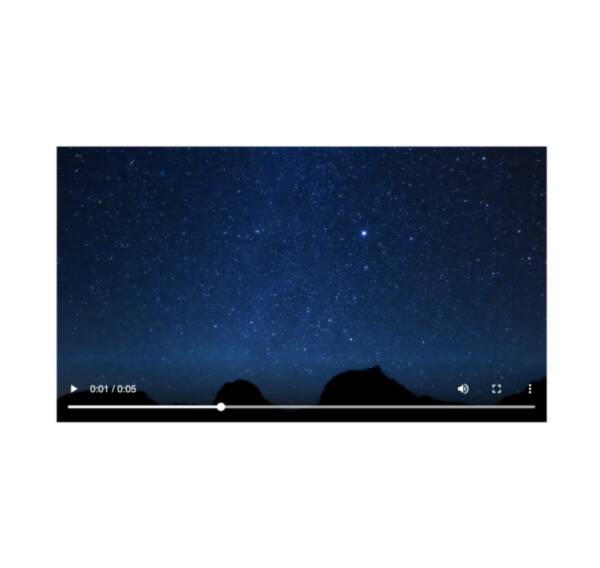
Video
Display a video player.
st.video(numpy_array)
st.video(video_bytes)
st.video(file)
st.video("https://example.com/myvideo.mp4", format="video/mp4")
Layouts and containers
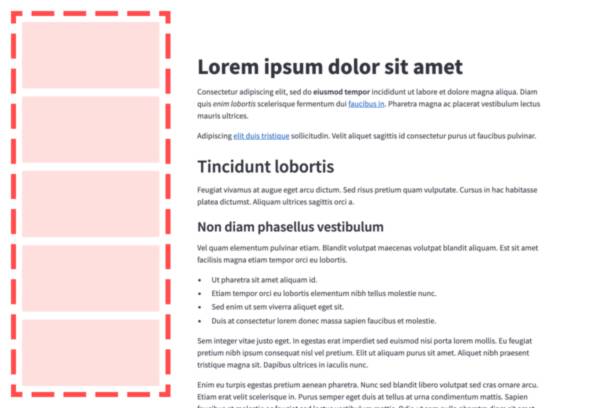
Sidebar
Display items in a sidebar.
st.sidebar.write("This lives in the sidebar")
st.sidebar.button("Click me!")
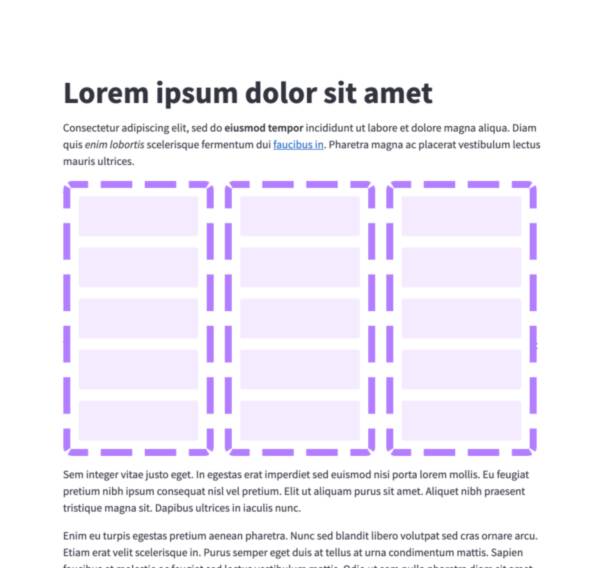
Columns
Insert containers laid out as side-by-side columns.
col1, col2 = st.columns(2)
col1.write("this is column 1")
col2.write("this is column 2")
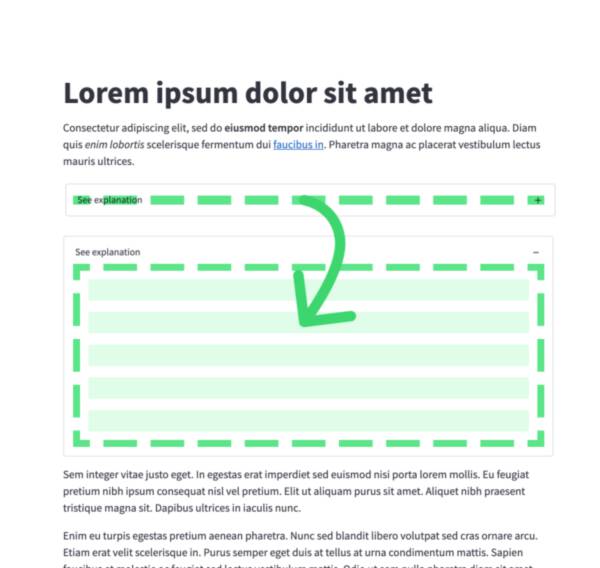
Expander
Insert a multi-element container that can be expanded/collapsed.
with st.expander("Open to see more"):
st.write("This is more content")
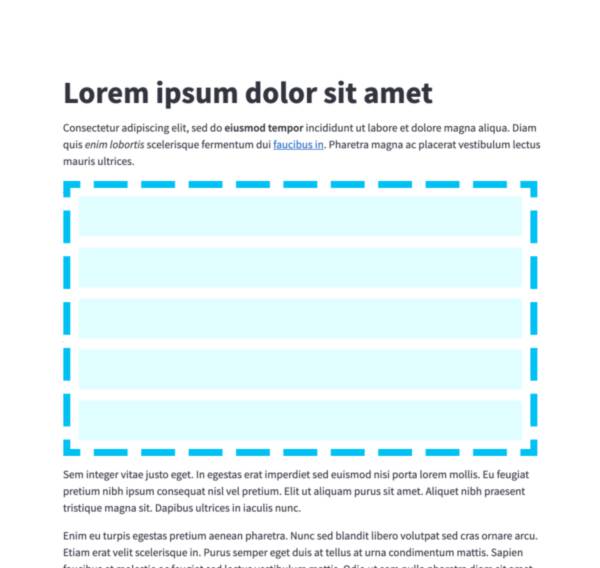
Container
Insert a multi-element container.
c = st.container()
st.write("This will show last")
c.write("This will show first")
c.write("This will show second")
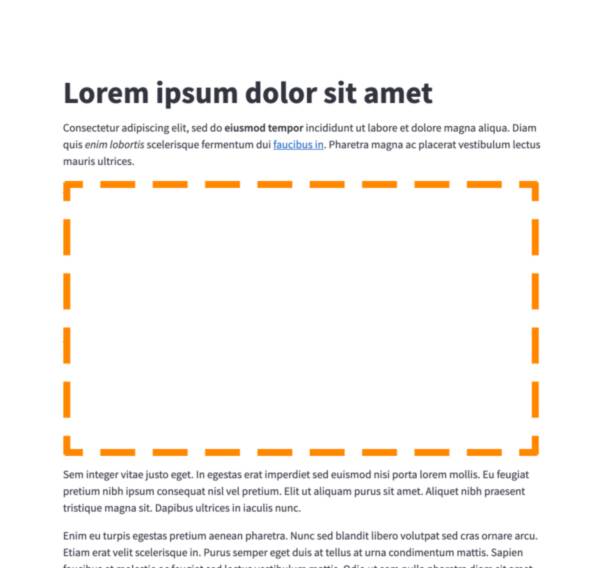
Empty
Insert a single-element container.
c = st.empty()
st.write("This will show last")
c.write("This will be replaced")
c.write("This will show first")
Display progress and status
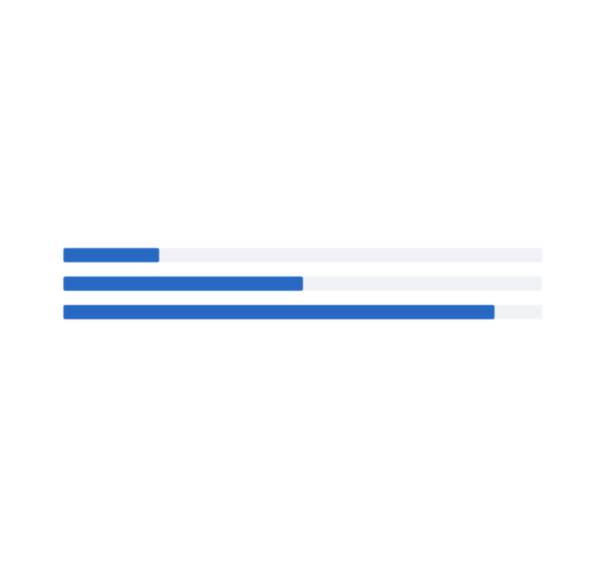
Progress bar
Display a progress bar.
for i in range(101):
st.progress(i)
do_something_slow()
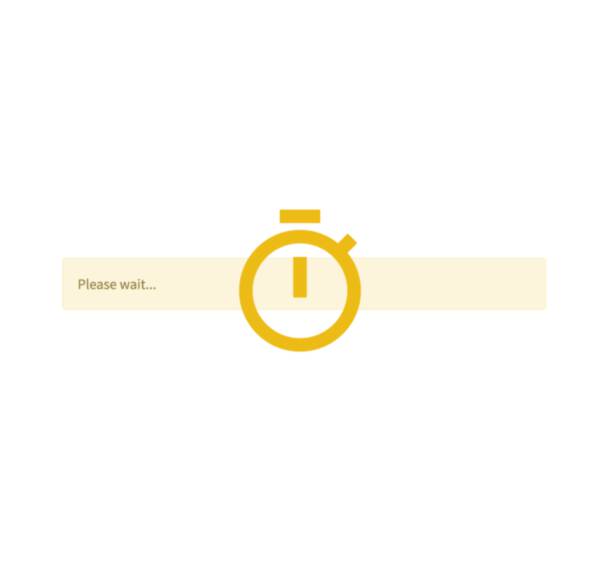
Spinner
Temporarily displays a message while executing a block of code.
with st.spinner("Please wait..."):
do_something_slow()
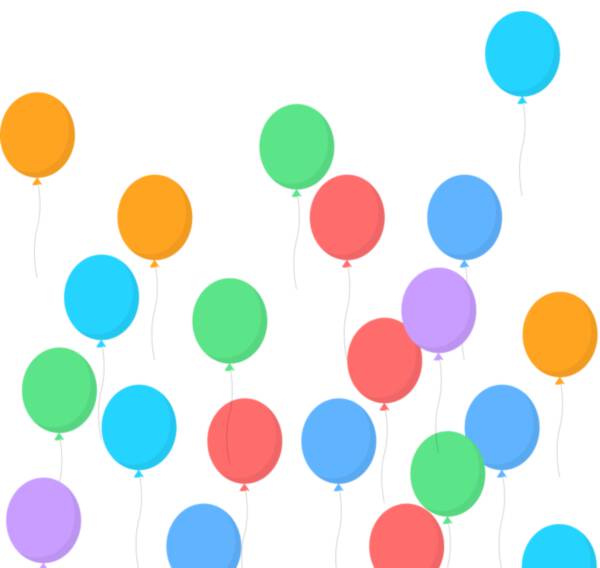
Balloons
Display celebratory balloons!
do_something()
# Celebrate when all done!
st.balloons()
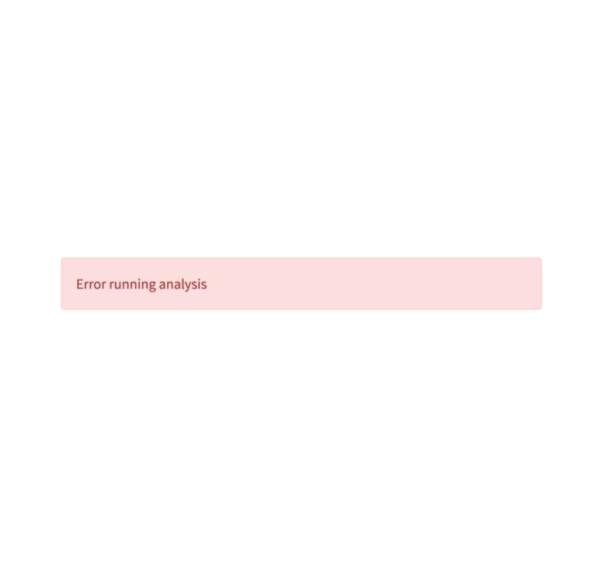
Error box
Display error message.
st.error("We encountered an error")
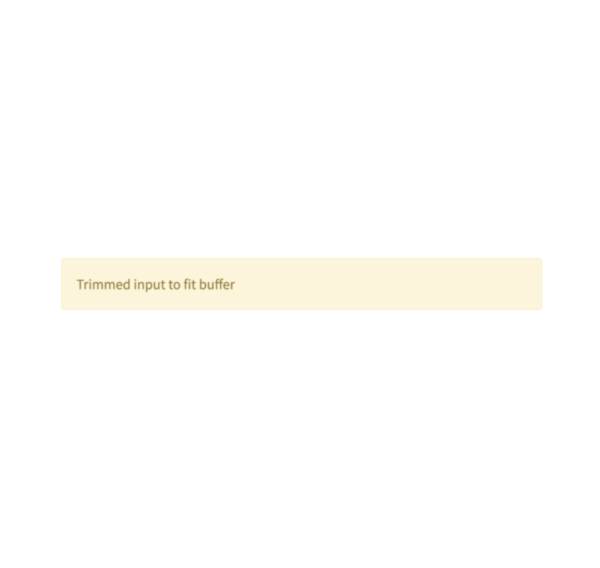
Warning box
Display warning message.
st.warning("Unable to fetch image. Skipping...")
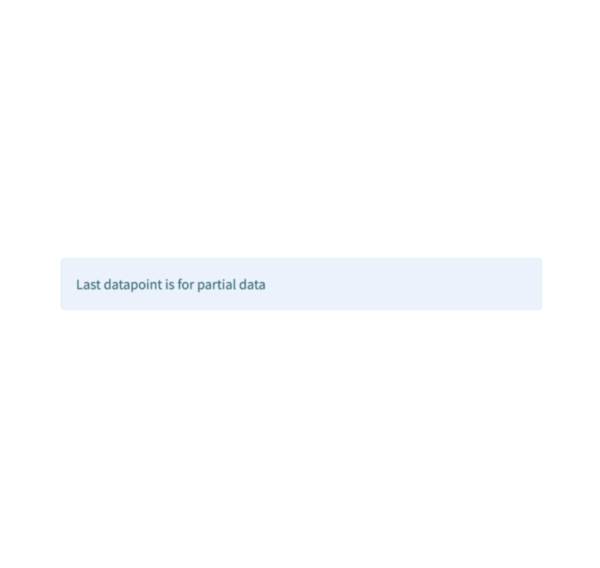
Info box
Display an informational message.
st.info("Dataset is updated every day at midnight.")
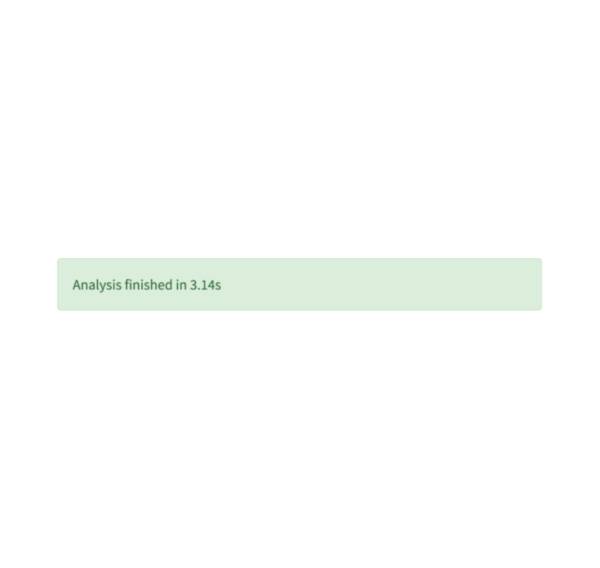
Success box
Display a success message.
st.success("Match found!")
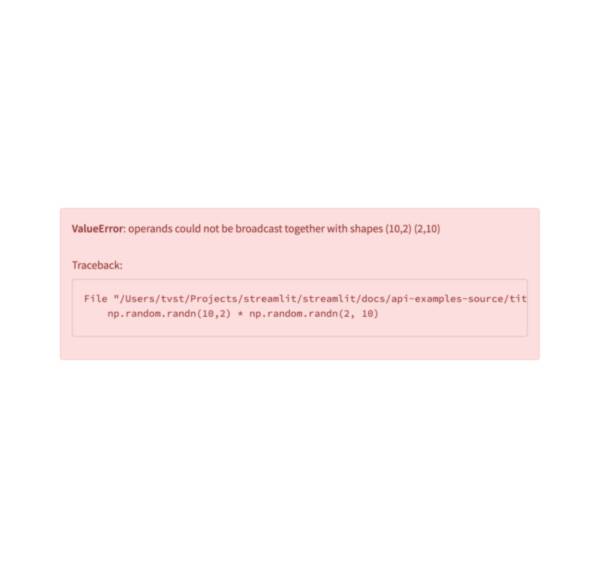
Exception output
Display an exception.
e = RuntimeError("This is an exception of type RuntimeError")
st.exception(e)
Control flow
Forms
Create a form that batches elements together with a “Submit” button.
with st.form(key='my_form'):
username = st.text_input("Username")
password = st.text_input("Password")
st.form_submit_button("Login")
Stop execution
Stops execution immediately.
st.stop()
Rerun script
Rerun the script immediately.
st.experimental_rerun()
Utilities
Set page title, favicon, and more
Configures the default settings of the page.
st.set_page_config(
title="My app",
favicon=":shark:",
)
Echo
Display some code on the app, then execute it. Useful for tutorials.
with st.echo():
st.write('This code will be printed')
Get help
Display object’s doc string, nicely formatted.
st.help(st.write)
st.help(pd.DataFrame)
st.experimental_show
Write arguments and argument names to your app for debugging purposes.
df = pd.DataFrame({
'first column': [1, 2, 3, 4],
'second column': [10, 20, 30, 40],
})
st.experimental_show(df)
Get query paramters
Return the query parameters that are currently showing in the browser's URL bar.
st.experimental_get_query_params()
Set query paramters
Set the query parameters that are shown in the browser's URL bar.
st.experimental_set_query_params(
show_map=True,
selected=["asia"]
)
Mutate charts
Add rows
Append a dataframe to the bottom of the current one in certain elements, for optimized data updates.
element = st.line_chart(df)
element.add_rows(df_with_extra_rows)
State management
Session state
Session state is a way to share variables between reruns, for each user session.
st.session_state['key'] = value
Performance
Caching
Function decorator to memoize function executions.
@st.cache(ttl=3600)
def run_long_computation(arg1, arg2):
# Do stuff here
return computation_output
Memo
Experimental function decorator to memoize function executions.
@st.experimental_memo
def fetch_and_clean_data(url):
# Fetch data from URL here, and then clean it up.
return data
Singleton
Experimental function decorator to store singleton objects.
@st.experimental_singleton
def get_database_session(url):
# Create a database session object that points to the URL.
return session
Clear memo
Clear all in-memory and on-disk memo caches.
@st.experimental_memo
def fetch_and_clean_data(url):
# Fetch data from URL here, and then clean it up.
return data
if st.checkbox("Clear All"):
# Clear values from *all* memoized functions
st.experimental_memo.clear()
Clear singleton
Clear all singleton caches.
@st.experimental_singleton
def get_database_session(url):
# Create a database session object that points to the URL.
return session
if st.button("Clear All"):
# Clears all singleton caches:
st.experimental_singleton.clear()